Adding Terms
You can configure your custom terminology provider to support adding and editing of terminology entries. Learn how to implement some simplified functionality for adding source and target terms to your delimited text list.
In Trados Studio, you can add source and target terms on the fly by marking them in the Editor and by then clicking either the Add New Term button, or the Quick Add New Term button.
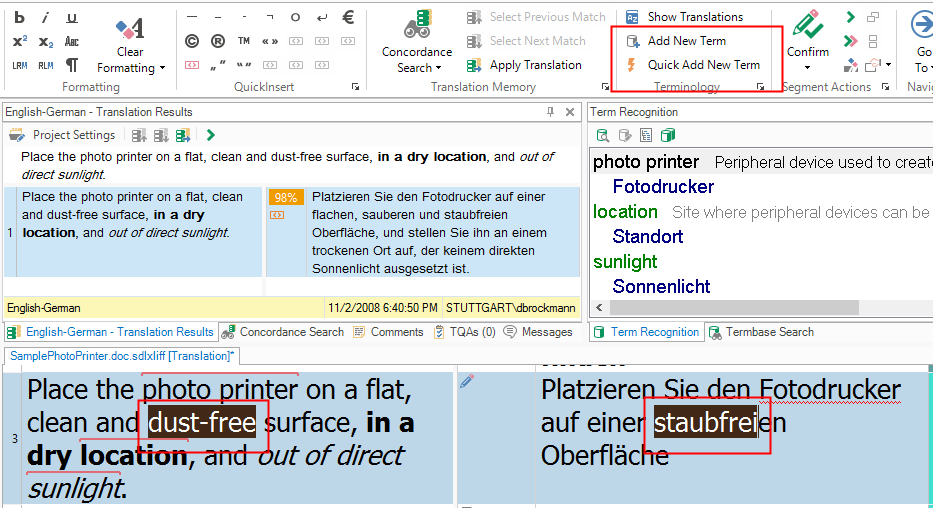
The difference between these two buttons in the standard, MultiTerm-based implementation of Trados Studio is that Add New Term does not immediately save the term pair to the termbase, but opens up an editor control in the Termbase Viewer window that allows you to make changes to the terms before saving them. Quick Add New Term adds the term pair directly to the terminology source and displays the newly-created entry in the Termbase Viewer window. Our simplified implementation will not offer any editing function. This means that the same thing will happen in our implementation, regardless of whether you click Add New Term or Quick Add New Term.
Open the MyTerminologyProviderViewerWinFormsUI.cs class and go to the AddTerm() function. Trados Studio passes the currently selected source and target term to this function through the source and target string parameters. We then implement the following functionality, which works like this:
- Open the glossary text file.
- Loop to the end of the text file, while counting the lines to determine the next entry id.
- Insert the new source and target term (with empty definition).
- Display the newly-created entry in the Internet Explorer control of the Termbase Viewer window.
#region "Enable term adding and editing
public bool CanAddTerm => true;
public bool IsEditing => true;
#endregion
#region "AddTerms"
//Triggered when the Studio user selects a source (and target) term, and then clicks the
//corresponding button for adding the term or term pair to the glossary.
//We loop through all existing term pairs to retrieve the next possible entry id,
//then we append the term or term pair to the text file.
public void AddTerm(string source, string target)
{
string fileName = _terminologyProvider.fileName.Replace("file:///", "");
StreamReader glossaryFile = new StreamReader(fileName);
int i = 0;
while (!glossaryFile.EndOfStream)
{
i++;
glossaryFile.ReadLine();
}
glossaryFile.Close();
int newEntryId = i + 1;
StreamWriter outFile = new StreamWriter(fileName, true);
outFile.WriteLine((newEntryId.ToString() + ";" + source + ";" + target + ";"));
outFile.Close();
//Show added entry in web browser control of the Termbase Viewer window
string tmpFile = System.IO.Path.GetTempPath() + "simple_list_entry.htm";
StreamWriter previewFile = new StreamWriter(tmpFile);
previewFile.Write("<html><body><b>Entry id:</b> " + newEntryId.ToString() +
"<br/><b>Source term:</b> " + source +
"<br/><b>Target term:</b> " + target +
"</body></html>");
previewFile.Close();
termControl.SetNavigation(tmpFile);
}
When a new source/target term pair has been added, the following will, for example, be displayed in the Termbase Viewer window:
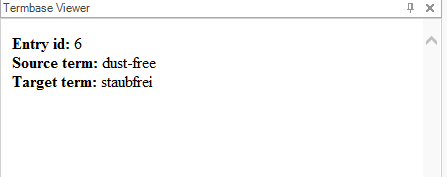
You can also implement your terminology provider to support editing. However, in this simplified example, we will not actually add any editing functionality. This would require us to implement an editing control in the Termbase Viewer window. All we are doing for the moment is to output a message in the AddAndEditTerm() function of the MyTerminologyProviderViewerWinFormsUI.cs class:
#region "AddEditTerm"
public void AddAndEditTerm(Entry term, string source, string target)
{
MessageBox.Show("Sorry, editing terms is currently not implemented :-(", "", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
#endregion
When you try to add a term that has already been added, Trados Studio throws the following message that prompts you to:
- Edit the entry by clicking Yes.
- Add the term again, thus risking creating a duplicate entry by clicking No.
- Abort the entire term add operation with Cancel.
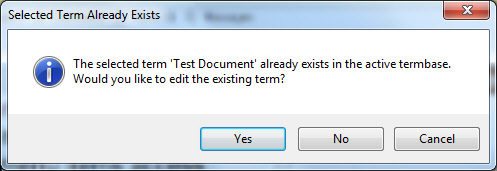
If you click Yes to edit the entry, the following message box will be displayed in our implementation and the Termbase Viewer window remains empty. In a 'real' implementation, the entry content will be shown in an edit control where you can still edit it.
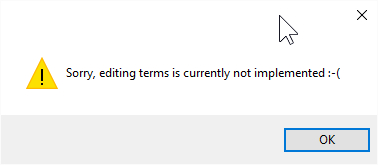